Vuejs Dynamic Slot Content
vue-table-dynamic
A dynamic table with sorting, filtering, editing, pagination, multiple select, etc.
Another really cool feature of VueJS is Dynamic Components. What are dynamic components you ask? Well, let’s say you have a main component and you would like to dynamically load other components into the main template by clicking a button or some other means. This is what can be accomplished with dynamic components.

- Slots are a powerful tool for creating reusable components in Vue.js, though they aren’t the simplest feature to understand. Let’s take a look at how to use slots and some examples of how they can be used in your Vue applications.
- Dynamic scoped slots in Vue.js Posted on 11 April 2019 in Web Slots are super-powerful in Vue.js. They let you inject components from a parent into a various parts of the child, helping you compose nice and generic components.
- Use v-if/v-else if the two components have very different slot content. Use dynamic components if they have the same slot content. I use dynamic components a lot to create different mobile and desktop interfaces.
- Vue.js - The Progressive JavaScript Framework. Modal Component Example. Features used: component, prop passing, content insertion, transitions.
vue-table-dynamic is a vue component of dynamic table. It's designed to respond to data changes in real time, and oriented to the runtime.
Features
- Multiple Select
- Search
- Sort
- Filter
- Pagination
- Edit
- Border
- Stripe
- Highlight
- Column Width
- Configure Header
- Fixed Header
Install
Usage
Import
Registration
Global registration
Local registration
Basic Table
Basic table usage
Border
Bordered table usage
border:
true
with borderborder:
false
without border
Stripe
Striped rows
stripe:
true
stripedstripe:
false
unstriped
Highlight
Highlighted rows/columns/cells
highlight:
{row?:Array<number>; column?:Array<number>; cell?:Array<[number,number]>;}
configure highlighted rows, columns, cells. such as:{row: [1], column: [1], cell: [[-1, -1]]}
if negative, the position from the end of the array.highlightedColor:
string
configure highlighted colors
Multiple Select
Select multiple rows
showCheck:
boolean
show checkbox of rowsgetCheckedRowDatas:
function
get data for all currently selected rowssetAllRowChecked:
function(selected:boolean)
toggle all selectionselect:
event
currently selected/unselected rows
Search
Filter rows by keyword
enableSearch:
boolean
enable/disable searchingsearch:
function(value:string)
manual row filtering
Sort
Sort rows based on specified column data
sort:
Array<number>
array members are sortable column indexes. such as:[0, 1]
Filter
Filter rows based on specified column data and rule
filter:
Array<{column:number; content:Array<{text:string; value:string number;}>; method:function;}>
specify filterable columns and rules. such as:[{column: 0, content: [{text: '> 2', value: 2}], method: (value, cell) => { return cell.data > value }}]
filter[].column:
column indexfilter[].content:
filter itemsfilter[].method:
filter rule.
Pagination
Table with pagination
pagination:
boolean
enable/disable paginationpageSize?:
number
row count of each page. default:10
pageSizes?:
Array<number>
options of row count per page. default:[10, 20, 50, 100]
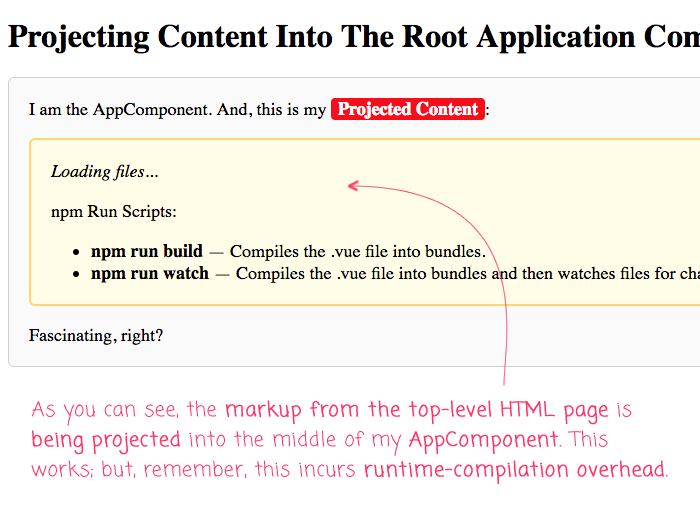
Edit
Editable table
Support specifying rows/columns/cells for editing
edit:
{row?:Array<number>; column?:Array<number>; cell?:Array<[number,number]>;}
configure editable rows, columns, cells. such as:{row: [1], column: [1], cell: [[-1, -1]]}
. if negative, the position from the end of the array.getData:
function()
table data changed after editing, get the latest data by this method.cell-change:
event
cell data changed eventedit:
{row: 'all'}
all cells can be edited- if
header
is'row'
, the first row is not editable
Column width
Vuejs Dynamic Slot Content Tool
Configure column width
columnWidth:
Array<{column:number; width:number string;}>
such as:[{column: 0, width: 60}, {column: 3, width: '15%'}]
columnWidth[].column
index of columncolumnWidth[].width
width of column. number for pixel value, string for percentage
Header Configure
header:
row
the first row is headerheader:
column
the first column is headerheader:
'
no header
Fixed Header
Vuejs Dynamic Slot Content Key
Fix header by configure the height of table
height:
number
table height- when the value of
header
is not'row'
, the first row is a normal row, will not fixed
API
Vuejs Dynamic Slot Content Guide
Attributes
params
is the object that need to be passed to the componentprops
- the following items are all child properties of the
params
object data
is required attribute, others are optional
name | description | type | optional value | default value |
---|---|---|---|---|
data | source data | Array<[number, ..., number]> | - | [] |
header | configure header | string | row : the first row is header; column : the first column is header; ' : no header | ' |
border | table with border | boolean | true /false | false |
stripe | striped table | boolean | true /false | false |
highlight | configure highlighted rows, columns, cells. such as: {row: [1] , column: [1] , cell: [[-1, -1]] }. if negative, the position from the end of the array. | {row?:Array<>; column?:Array<>; cell?:Array<>;} | - | {} |
highlightedColor | highlighted colors | string | - | #EBEBEF |
showCheck | show checkbox of rows. Only when the header is 'row' , the first cell of the first row is the checkbox of all rows. Otherwise, the first cell is the checkbox of the first row | boolean | true /false | false |
enableSearch | enable/disable searching, filter rows by keyword | boolean | true /false | false |
minWidth | min width of table | number | - | 300 |
maxWidth | max width of table | number | - | 1000 |
height | table height. fix header by configure the height of table | number | - | - |
rowHeight | row height | number | >= 24 | 30 |
columnWidth | Configure column width | Array<{column:number; width:number/string;}> | - | - |
sort | sort rows based on specified column data | Array<number> | - | - |
filter | filter rows based on specified column data and rule. column : index; content : filter items; method filter rule. | Array<{column, content, method}> | - | - |
edit | specifying rows/columns/cells for editing. table data changed after editing, get the latest data by getData method | {row?:Array<>; column?:Array<>; cell?:Array<>;} | - | - |
pagination | table with pagination | boolean | true /false | false |
pageSize | row count of each page | number | - | 10 |
pageSizes | options of row count per page | Array<number> | - | [10, 20, 50, 100] |
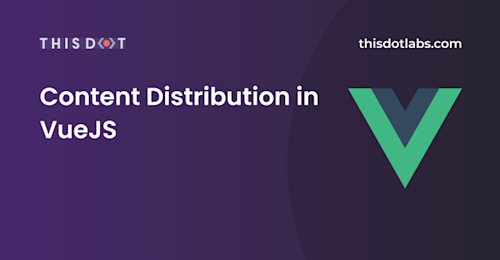
Methods
method name | description | parameters | return |
---|---|---|---|
getData | table data changed after editing, get the latest data by this method | - | Array<[number, ..., number]> |
getCheckedRowDatas | get data for all currently selected rows | includeWhenHeaderInfirstRow: boolean include header row when the first row is header,default is false | Array<[number, ..., number]> |
getRowData | get row data by index | rowIndex:number index;isCurrent: boolean is the index sorted,default is false | Array<number> |
search | manual row filtering | searchValue:string keyword | - |
clearSearch | clear searching, show all rows | - | - |
Vuejs Dynamic Slot Content Tutorial
Events
event name | description | parameters |
---|---|---|
select | event when selecting a row | checked: boolean ; index: number ; data: Array<stringnumber> |
select-all | event when clicking the checkbox in table header | isCheckedAll: boolean |
row-click | event when clicking a row | index:number ; data:Array<stringnumber> |
cell-click | event when clicking a cell | rowIndex:number ; columnIndex:number ; data:stringnumber |
cell-change | event when edting a cell | rowIndex:number ; columnIndex:number ; data:stringnumber |
sort-change | event when sorting | index: number ; value: string |